What are front-end frameworks? Front-end frameworks are software development tools used by custom web application development companies for building user interfaces. They provide a collection of pre-written code that can be reused by following specific standards, which typically include conventions for organizing different parts of the code. By adhering to these standards, front-end frameworks help developers understand and work with code written by others more easily, making them especially useful when different dedicated developers are working on the same codebase. This ensures that your front-end developers apply a single set of rules, documentation, and best practices to write code. If you lose one of the developers, it's easier to find a replacement without much onboarding time, as every developer immediately understands how to do the same kind of work in the same way.
If not using a Front-End Framework, then What?
There are a few alternatives to using a front-end framework for building the user interface of a web application.
One option is to use vanilla or pure JavaScript and build the UI from scratch using just the core language features and APIs.
Another option is to use a library like jQuery, which provides a set of useful functions for working with the DOM and making AJAX requests.
Here are a few more options for building the user interface of a web application without using a front-end framework:
- Server-side rendering. This involves generating the HTML for a web page on the server, rather than in the client's browser. This can be done using a server-side language like PHP, Ruby, or Python, and can be a good option for applications that do not require a lot of client-side interactivity.
- CSS frameworks. This type of front-end framework provides a set of styles, layout patterns, and design principles for building web interfaces. They are typically focused on providing a consistent look and feel for web applications, and can include styles for common UI elements such as buttons, forms, tables, and more. CSS frameworks can be a useful tool for building the visual aspect of a web application, and can be especially helpful for quickly prototyping or building simple applications.
- Static site generators. These are tools that can generate static HTML, CSS, and JavaScript files from templates and data sources. They can be a good option for building simple websites or web applications that do not require a dynamic, real-time update of the content.
- No-code platforms. There are a number of platforms that allow you to build web applications without writing any code. These platforms typically provide a set of pre-built templates and components that you can customize and arrange to create your application. They can be a good option for non-technical users or for prototyping ideas quickly.
- Hybrid approaches. You can also use a combination of different tools and technologies to build the user interface of a web application. For example, you could use a static site generator to build the basic structure of the application, and then add some interactivity using pure JavaScript or a library like jQuery.
JavaScript Front-End Frameworks vs pure JavaScript
Why use a front-end Javascript framework? The most commonly used programming language is JavaScript. And the most popular front-end frameworks are JavaScript-based: React (officially, it’s a library), Angular, or Vue.js
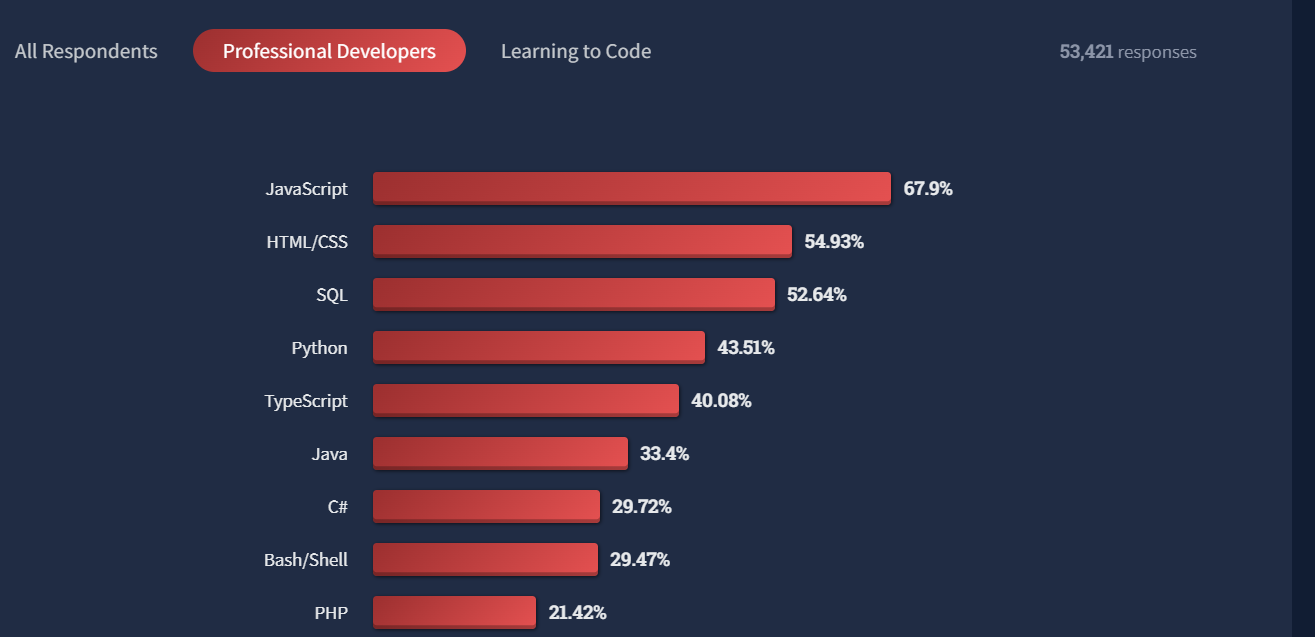
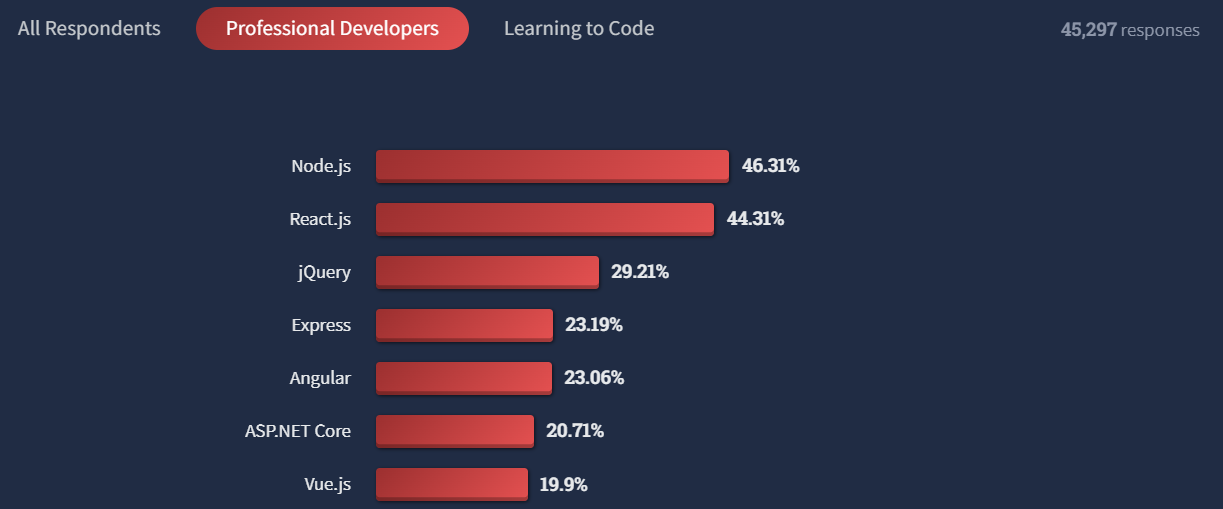
https://survey.stackoverflow.co/2022
By using pure JavaScript instead of a front-end framework, a developer must create their own structure for organizing and implementing features in their applications. While this can be sometimes a more flexible approach, it can also be more time-consuming and require more effort to maintain the codebase as the application grows and evolves.
Some key differences between JavaScript front-end frameworks and pure JavaScript include:
- Code reusability. Front-end frameworks often provide a set of reusable components so developers don’t have to rebuild them from scratch. React, for example, allows the use of reusable UI components, reducing development time. Conversely, pure JavaScript developers need to create their own code reuse mechanisms if necessary.
- Code maintenance. Front-end frameworks provide a consistent structure and set of conventions for organizing and implementing code to make it easier for developers to understand and work with code written by others, and reduce the risk of errors or bugs. Pure JavaScript developers must create their own conventions and patterns for organizing and implementing code.
- Easy-scalable functionality. Front-end frameworks provide out-of-the-box key features of every complex web application such as routing. Pure JavaScript developers must implement them manually.
- Performance. Front-end frameworks are designed to enhance the rendering and performance of the front end of web applications. React, for example, offers specific strategies for performance optimization. Pure JavaScript may not offer the same level of performance and scalability.
- Community and ecosystem. Front-end frameworks typically have a large and active community of developers and a wide range of resources and tools available, including documentation, tutorials, and third-party libraries. This can make it easier to find support and resources when working with a front-end framework. Pure JavaScript, as a core programming language, also has a large and active community, but it may not have the same level of resources and tools specifically tailored for building the front-end of web applications.
Features of Front-end Frameworks
Components Functionality
Most frameworks have a reusable set of commonly-used components to construct a UI fast.
For example, AuthorCredit component (React) can be used while building a blog or online magazine. It allows displaying a portrait of the author and a short byline about them for each article.
And matAutocomplete component (Angular) is useful when you need to show a list of auto-complete options when the user tries to type something in the field.
The possibility to quickly build and re-use custom UI components is a distinguishing feature of a modern front-end framework.
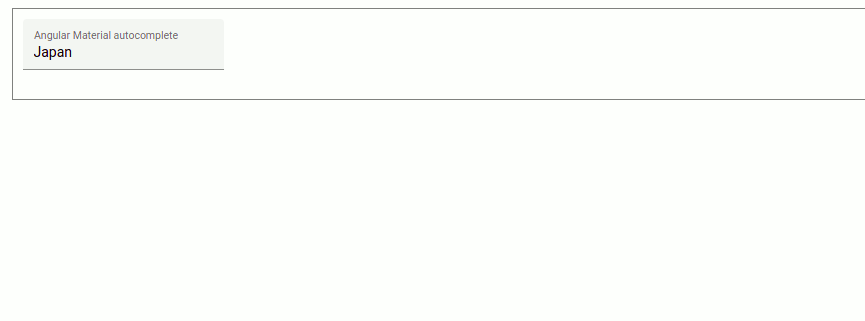
matAutocomplete Angular component
State Management
State management is a crucial aspect of front-end development, as it allows components to track and update their own data values without the need for additional code.
A front-end framework's built-in state-handling mechanism makes this process easier by providing tools like the useState() hook or libraries like Redux, XState, and Mbox. These tools, given an initial data value, automatically keep track of and update that value as needed.
Here is an example of how state management is generally easier to implement using a front-end framework compared to using pure JavaScript.
Consider a simple front-end application that displays a list of items, and allows the user to add and remove items from the list.
Using pure JavaScript, the developer would need to write code to handle the following tasks:
- Display the list of items.
- Add a new item to the list when the user clicks a "Add" button.
- Remove an item from the list when the user clicks a "Remove" button.
- Update the display of the list to reflect the current state of the list.
To implement these tasks using pure JavaScript, the developer would need to write code to manually update the DOM elements that display the list of items whenever the state of the list changes. This would require a significant amount of code, and could be prone to bugs and maintenance issues.
On the other hand, using a front-end framework like React or Vue, the developer can manage the state of the list in a more efficient way.
For example, the developer could use a state management tool provided by the framework to update the state of the list whenever the user clicks the "Add" or "Remove" button, and the framework would automatically update the display of the list to reflect the current state of the list. This can save a significant amount of time and effort compared to writing all of the code from scratch using pure JavaScript.
Browser Events Handling
In addition to monitoring ongoing state, front-end frameworks also react to events that occur in the browser. Examples of these events include closing the browser window, a web page finishing loading, a form being submitted, and errors occurring.
The mechanism behind event handling can be described as follows: "listener code listens for the event to occur, and the handler code runs in response to it happening."
Some examples of such events include:
- Form events, such as "submit", "reset", "focus", "blur", and "change". These events are fired when a form is submitted, reset, or its elements receive or lose focus, or the value of a form element changes.
- Mouse events, such as "click", "dblclick", "mousedown", "mouseup", "mouseover", "mouseout", "mousemove", and "contextmenu". These events are fired when the user interacts with the mouse, such as clicking on an element or moving the mouse over an element.
- Keyboard events, such as "keydown", "keyup", and "keypress". These events are fired when the user interacts with the keyboard, such as pressing or releasing a key.
- Window events, such as "resize", "scroll", "beforeunload", and "error". These events are fired when the window is resized, scrolled, about to be closed, or an error occurs.
Front-end frameworks like React, Angular, and Vue.js provide a range of tools and libraries that can make it faster and easier to implement event handling for these and other browser events compared to using pure JavaScript.
For example, they provide declarative syntax, automatic event listener management, and reactive data binding, which can help the developer focus on the business logic of the application rather than on the low-level details of DOM manipulation.
Here are some points in more detail.
Declarative syntax
Front-end frameworks provide a declarative syntax that allows the developer to specify the desired behavior of the application in a more abstract and concise way, rather than writing imperative code to manipulate the DOM directly.
For example, in the React example, the useEffect() hook is used to attach and remove the "submit" event listener to the form element in a declarative way, rather than using the imperative addEventListener() and removeEventListener() methods.
Automatic event listener management
Front-end frameworks can automatically manage the lifecycle of event listeners, attaching them when the component is mounted and removing them when the component is unmounted. This can help the developer avoid memory leaks and other issues that might arise from attaching and removing event listeners manually.
For example, in the Angular example, the HostListener decorator is used to attach the "resize" event listener to the window object, and the Angular framework takes care of removing the listener when the component is destroyed.
Reactive data binding
Front-end frameworks provide reactive data binding, which allows the developer to specify how the UI should change in response to data changes. This can make it easier to handle events that involve updating the UI, as the framework can automatically update the DOM based on the changes in the data model.
For example, in the Vue.js example, the "scroll" event listener is attached to the document object, and the Vue.js framework takes care of updating the DOM based on the changes in the data model caused by the "scroll" event.
Client-Side Rendering
Client-side rendering is often used to update parts of the UI without requiring a full page reload by using such concepts as, for example, Virtual DOM.
For example, if a user adds an item to their cart in an e-commerce application, the client-side rendering process could be used to update the display of the cart icon to show the correct number of items, update the cart page to show the correct list of items, and potentially update other areas of the UI as well (e.g., updating the total cost of the items in the cart).
The Virtual DOM is a lightweight in-memory representation of the actual Document Object Model (DOM) that is used to update the actual DOM in an efficient way. When a user interacts with a web application, the front-end framework compares the previous and current versions of the Virtual DOM and calculates the minimum number of changes needed to update the actual DOM. This process is called "reconciliation" or "diffing".
By using the Virtual DOM, front-end frameworks can significantly improve the performance of a web application, as it reduces the number of costly DOM manipulations that need to be performed. This can be especially beneficial for complex front-end applications that require frequent updates to the UI.
While pure JavaScript does not include the Virtual DOM as a built-in feature, it is possible to use pure JavaScript to implement the Virtual DOM concept. However, this would require writing a significant amount of code to handle the various aspects of the Virtual DOM, such as diffing and reconciliation, and could be time-consuming to implement and maintain.
Form Processing
Front-end developers have to create forms for almost every project. And it’s surprisingly tedious to do.
The process involves a lot of repetitive work such as creating input elements, adding field labels, and help texts, field grouping, performing form validation, creating custom validation rules, modifying default validation messages, forms theming, and much more.
Front-end frameworks have libraries that make form building and validating much easier.
Here is an example of why form processing is generally better to do with a front-end framework compared to using pure JavaScript:
Consider a simple form that allows a user to enter their name and email address, and then submit the form to send the data to the server.
Using pure JavaScript, the developer would need to write code to handle the following tasks:
- Display the form fields and submit button. The developer can use pre-built UI components provided by the framework to display the form fields and submit button.
- Validate the form input. The developer can use form validation tools provided by the framework to check that the name and email fields are not empty and that the email field is a valid email address.
- Display error messages if the form input is invalid. The developer can use the form validation tools provided by the framework to display error messages if the form input is invalid.
- Send the form data to the server when the submit button is clicked. The developer can use an HTTP library provided by the framework, or make an HTTP request using pure JavaScript, to send the form data to the server when the submit button is clicked.
- Display a success or error message depending on the server's response. The developer can use the framework's UI components and state management tools to display a success or error message depending on the server's response.
Client-Side Routing
There are also several reasons why using a front-end framework can be a better choice for implementing client-side routing than using pure JavaScript.
- Advanced features. Front-end frameworks usually provide a range of advanced features for client-side routing, such as route-level code splitting, lazy loading, navigation guards, transition animations, and more. These features can make it easier to implement complex routing scenarios and provide a better user experience for the application.
- Ecosystem. Front-end frameworks usually have a large and active community of developers, which can provide a wealth of resources, tools, and libraries for working with client-side routing. This can make it easier to find solutions to common routing problems and to integrate the routing mechanism with other libraries or APIs.
- Maintenance. Front-end frameworks often provide a unified and consistent API for working with client-side routing, which can make it easier to maintain the routing code over time. This is especially useful if the application has a large number of routes and requires frequent updates to the routing mechanism.
- Reusability. Front-end frameworks often provide reusable components that can be used across different routes, which can make it easier to build and maintain a consistent and coherent UI for the application. This can save time and effort compared to building the UI from scratch for each route using pure JavaScript.
Errors Handling and Reporting
Unlike the backend, frontend code doesn't run on a single platform but on dozens of browsers and device types. At the same time, users almost never report UI glitches, slow performance, and broken interfaces, they just leave with a bad impression.
Businesses want their web apps to remain working even if a front-end error occurs, and, in the worst cases, show readable and understandable messages to users.
The support team also should get the error code to resolve issues ASAP.
It’s easier to handle and report front-end errors using appropriate frameworks. For example, libraries like React-Error-Boundary can help write less code for this purpose.
Frameworks provide mechanisms like tracking errors centrally (mostly for unexpected errors) with error handlers and their functionality easily may be extended by third-party bug tracking and monitoring solution.
With error handling and reporting tools, located centrally, developers may scale the app or modify the error handling through one file and update it globally.
Facilitation of Tests Writing
Frontend testing focus on the validation of menus, forms, buttons, and other web application elements visible to end users. Tests check out how quickly elements load, what are their response times to user actions, and so on.
Front-end frameworks have extensive testing tools with capabilities from unit to integration testing.
Test coverage ensures your web software continues to behave in the way that you'd expect and gives you confidence in your UI code.
Using a JavaScript front-end framework can facilitate test writing compared to using separate libraries or vanilla JavaScript or jQuery because it provides a structured and consistent approach to testing.
Here is an example of how you might write tests for the login form using vanilla JavaScript or jQuery, and how this approach may not be as structured and consistent as using a JavaScript front-end framework.
To test the login form using vanilla JavaScript or jQuery, you would need to manually create the form elements and add them to the DOM (Document Object Model). You could then use JavaScript or jQuery functions to simulate user actions and verify the form's behavior.
This approach may not be as structured and consistent as using a JavaScript front-end framework, because you are responsible for manually creating and manipulating the DOM elements and handling the testing process yourself. This can make it more difficult to write and maintain tests, as you need to manage the details of the testing process manually.
By contrast, using a JavaScript front-end framework such as React, Angular, or Vue.js provides a more structured and consistent approach to testing, as it includes built-in tools and features for rendering and interacting with components in a test environment.
When to Use a Front-end Framework
For building Single-Page Applications
Single-Page Applications are those ones that use an architecture where data updates and navigation occur without page reloading. For example, dashboard apps.
The best way to build such applications, where rich interactivity, deep session depth, and non-trivial stateful UI logic are required, is by using front-end frameworks.
For building Server-Side Rendering Applications
When the initial load performance for the app is absolutely critical or when your web app is sensitive to SEO, server-side rendering is applied.
For such cases, the front-end frameworks provide APIs that allow utilizing a server to generate an HTML page for users without the necessity to see the white screen, while the browser loads JavaScript files.
Server-side rendering greatly improves Core Web Vital metrics such as Largest Contentful Paint (LCP).
To speed up your site's performance even further, static-site generation techniques, also known as JAMStack, are used. Front-end framework helps pre-render an entire application into HTML and serve them as static files.
Let’s build an interactive and responsive UI that your app users will love. Our front-end developers apply best practices to write top-quality code and deliver the look and functionality you expect rapidly and within a budget.
Rate this article
Our Clients' Feedback
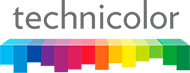
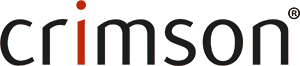
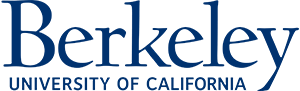



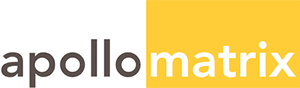
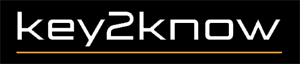
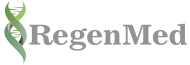
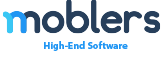

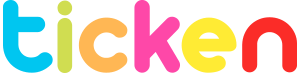
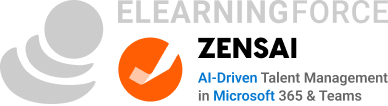
Belitsoft has been the driving force behind several of our software development projects within the last few years. This company demonstrates high professionalism in their work approach. They have continuously proved to be ready to go the extra mile. We are very happy with Belitsoft, and in a position to strongly recommend them for software development and support as a most reliable and fully transparent partner focused on long term business relationships.
Global Head of Commercial Development L&D at Technicolor