As your application complexity increases, React's component-based architecture becomes more important for managing it efficiently. It allows you to tackle the complexity by focusing on individual pieces instead of the entire UI. Components are key in React's design, enabling developers to create reusable and maintainable interfaces.
What is a React Component
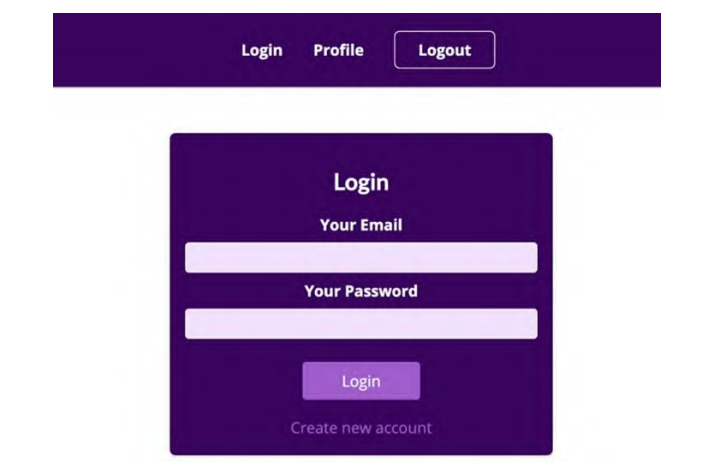
Structure of React Components
A React component is a JavaScript function that defines part of the user interface. It returns JSX to describe what to render and uses imports and exports to interact with other components.
Absolutely necessary parts
Function
To create a React component, you must define a JavaScript function. This is the fundamental building block.
Not all functions are components. React components need to return something that React can render on the screen.
For example, if a function returns a plain JavaScript object, React doesn't know how to display it by default. You need to format and display the data. You can render JSX code by default, but you can also return strings, numbers, or arrays that contain JSX elements, strings, or numbers.
Our React development company has expert teams that create modern, interactive user interfaces with optimal performance and scalability. Get in touch for seamless integration, fast loading times, and captivating visuals for your projects.
JSX
A React component must ultimately return a JSX syntax extension to JavaScript that describes the UI it represents. Without this, there's nothing to render. React transforms HTML-like JSX code to React.createElement calls under the hood.
You can write React components entirely using React.createElement calls, but JSX is generally preferred for its readability and natural feel when defining UI structures. In rare cases, if you need highly specialized element configurations that are difficult to achieve with JSX, direct function calls can offer more flexibility.
It's important to understand that JSX is not directly sent to the browser. React acts as an interpreter, transforming your JSX into browser-friendly instructions to change the actual web page (the DOM).
This means that certain assumptions about standard HTML may not always apply in a JSX context, which can prevent bugs and confusion.
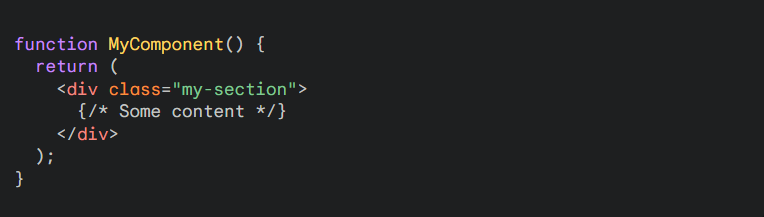
You might think: "I have a CSS class called my-section, this should style the div." In reality, the class attribute won't work in JSX. It needs to be className.
The reason for using className instead of class in React is because class is a reserved keyword in JavaScript. Since React uses JSX, which is an extension of JavaScript, we must use className instead of the class attribute.
Moreover, it's easy to assume you're writing regular HTML tags. However, div, h1, p, etc., are actually stand-ins for React components that come built-in with the React library (specifically, the react-dom package).
JSX isn't a standard part of JavaScript. Your React project setup includes tools (like Babel) to transform it during development or the build process.
JSX also lets you embed JavaScript logic and expressions within your UI definitions. Curly braces { } within JSX mark places where you can directly insert JavaScript expressions. You can include variables, function calls, calculations – anything that results in a single value when evaluated. Variables and function calls inside the curly braces can be tied to your application's state or incoming data.
Since JSX elements are like any other JavaScript value, you have flexibility:
- Store JSX elements in variables for later use.
- Pass JSX elements as arguments to other functions or components.
JSX requires self-closing tags, even for elements that are traditionally "void" in HTML.
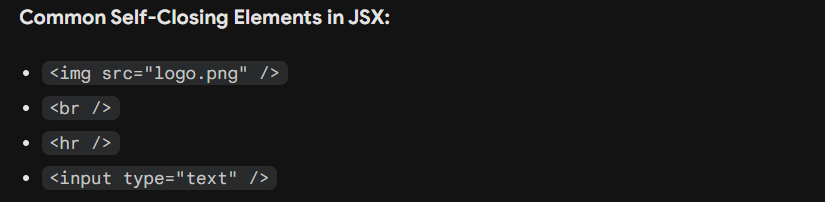
Optional (but extremely common and powerful) parts
Exports
Components in React can be exported and imported for other parts of your application. If a component is only used within the same file, it doesn't need to be exported.
State Management (using Hooks)
Components can be purely presentational and not manage their own state.
Event Handler Function
Events can be handled inline within JSX, but a separate function can help with organization, especially for more complex event logic.
Conditional Rendering
Conditional element rendering is not required for a component to be dynamic.
Other Features of Components
Components are reusable
Components are like LEGO bricks for your webpage. They are independent pieces of code that define how a specific section of your user interface (UI) should look and function.
The great thing about components is that you can reuse them throughout your application. For example, a button or form component can be created once and used in multiple places.
Components can nest within other components
This allows you to break down complex UIs into smaller, more manageable units. For example, a navigation bar component could contain individual button components for each link.
Think of your React application as a tree of components. At the very top is the root component (often named App), and other components branching out from it.
React's role is to traverse this component tree, call each component's function, and translate the returned JSX into instructions that the browser can understand to creating or updating elements in the browser's DOM (Document Object Model, which is the actual structure of the webpage).
Naming conventions in React
To distinguish React components from regular JavaScript functions, it is a convention to use capitalized component names.
Using PascalCase (e.g., SubmitButton, UserProfile) for your custom React component names is a strongly recommended practice. When using your custom components within JSX, you must use an uppercase starting letter.
While using a components folder is common, the specific placement within your src folder is customizable. These are the two most common file naming approaches:
- PascalCase. Mirrors the component name (SubmitButton.js).
- Kebab-case. All lowercase, hyphen-separated (submit-button.js)
Class-based Components vs Functional Components
React expects components to be functions or classes.
The modern approach in React development is to use functional components and Hooks to build React components.
The addition of Hooks in React 16.8 allows functional components to do everything that class-based components can do, such as managing state and accessing lifecycle events.
Class-based components are becoming less common as functional components provide a cleaner way to build React applications.
React Component Splitting
There's no perfect formula for determining when to split components. It's a bit of an art that you'll develop with experience.
However, here are some guiding principles.
Reusability
Can a portion of your UI be used in multiple places within your application? If so, creating a standalone component makes it easier to reuse the same UI structure with different data.
Single Responsibility Principle
Does the component have a clear, focused purpose? Components become easier to understand and maintain when they each are responsible for one primary aspect of your UI.
Manageability
Is the component's code becoming too large or complex to easily read and reason about? Breaking it down into smaller, more focused components can improve maintainability.
Component-Driven Development
In component-based development, we create small, self-contained pieces of interface to build UIs. These components handle visuals, interaction, and functionality for specific use cases in the app.
Extracting components establishes a common language between designers and developers, improving collaboration and communication. This leads to smaller, maintainable code with reusable components for consistency and a better user experience.
The component-driven approach has benefits such as improved communication, predictable development timelines, and easier UI redesigns. By encapsulating code in small, reusable modules, we separate concerns and maintain a clean code organization. Components also lead to a consistent user experience throughout the app, simplifying UI redesigns and enhancements.
Switching to component-based development requires commitment from developers and designers, as it involves a shift in workflow and mindset. However, with a good component gallery, teams can quickly create new screens and maintain a maintainable codebase.
See how React.js helped us build a tailored LXP for corporate training. Our solution resolves key learning challenges, including personalized learning paths, real-time progress tracking, and gamification. Gain insights in our case study.
Components and Props
Splitting components introduces the need to make them configurable. This is where props come in. Props are React's way of passing data from a parent component into a child component, allowing customization without hardcoding values.
Props are like "attributes" for your custom components. You define what props a component expects to receive.
With props, parent components can pass data to a child component, customizing how it looks or behaves.
Instead of embedding values directly in your components, you can use props to make the output dynamic by using variables.
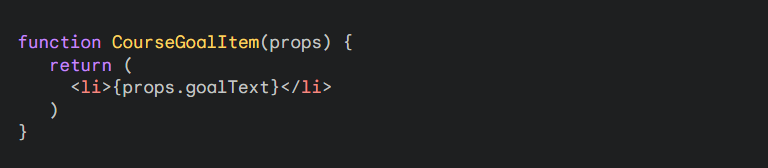

Let's unpack how props work in React components.
Passing props to components
Think of passing props as similar to setting attributes on HTML elements. You add attribute-like key-value pairs to your custom components in JSX.
You can also pass data as content between the opening and closing tags of a component, just like you would with HTML.
Consuming props in a component
React automatically provides a special props object as an argument to your component functions. It doesn't matter how you name this argument, but the convention is to use props.
The props object holds the key-value configuration data you passed in when using the component. You access the individual values using dot notation (e.g., props.title, props.id).

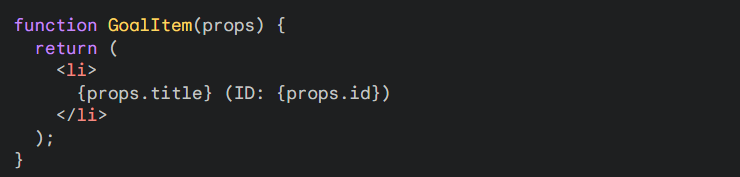
Use props when you want to configure a component from the outside, for instance from its parent component.
Use props.children when you want to pass content directly inside your component's tags. This is useful for components that act as wrappers or containers.
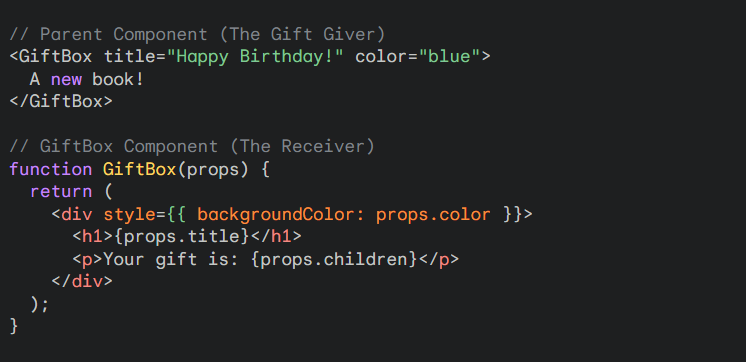
When components need props in React
You don't have to use props in every component.
The primary reason to use props is to make components more flexible and reusable by passing in different data. You can use the same component multiple times, customizing how it looks or behaves by changing the props you give it.
Example of a component that needs props
- A "Button" component that can have different labels (Login, Submit, etc.) would need a prop to pass in the button text.
- A "WelcomeMessage" component that displays a user's name would need a prop to receive the name.
Example of a component that might not need props
- A "Logo" component that always displays the same image.
- A "Timer" component that manages its own timing without external input.
How to handle multiple props in React components
You can add attributes directly to the component's opening tag, with each attribute representing a single prop. This is useful when you have a small number of well-defined props.

You also can create a JavaScript object that holds all the data (properties) you want to pass to the component. This object is then passed as a single prop. The best use case for this is when you have a large number of props, or when the props are logically related and make sense to group together.

Direct access using props.propertyName is the most straightforward way to access a specific prop's value within your component, where propertyName is the name of the prop you want to use.

Object destructuring JavaScript feature allows you to unpack properties from an object and assign them to individual variables. In the context of React components, you're destructuring the props object.

Spreading props in React
Sometimes you want to create a custom component that wraps another component (built-in or third-party) to add custom styling, logic, or behavior.
By creating a wrapper component, you lose the ability to directly pass props to the wrapped component. The wrapper component acts as an intermediary and has to explicitly define and handle all the props that should be passed down to the wrapped component.
Instead of manually listing all the props your component should accept, you can use the spread operator {...object}.
Prop drilling or Prop chains in React applications
As React applications grow more complex, components often end up being nested within other components.
Sometimes, data (in the form of props) needs to be passed from a parent component down to a nested child component several levels deep.
This process of passing props from a higher-level component through multiple intermediate components (that don't need those props themselves) to a deeply nested component is known as "prop drilling" or "prop chains".
As the application grows, prop drilling can become tedious, make the code harder to maintain, and cause performance issues.
Components that don't need certain props still have to accept and forward them, cluttering the code.
React provides alternative solutions to avoid excessive prop drilling, such as using context or state management libraries.
Enhance your application's advantages with personalized React development solutions. Contact us to hire offshore dedicated development team for expert React guidance.
Recommended posts
Our Clients' Feedback
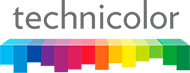
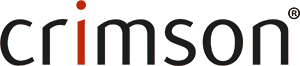
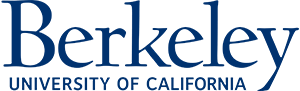



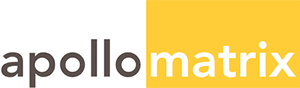
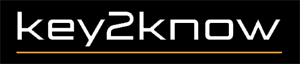
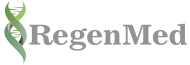
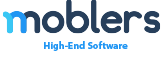

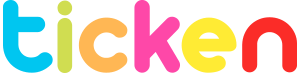
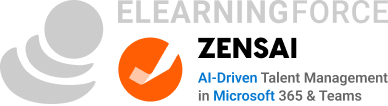
Belitsoft has been the driving force behind several of our software development projects within the last few years. This company demonstrates high professionalism in their work approach. They have continuously proved to be ready to go the extra mile. We are very happy with Belitsoft, and in a position to strongly recommend them for software development and support as a most reliable and fully transparent partner focused on long term business relationships.
Global Head of Commercial Development L&D at Technicolor