You choose a front-end framework for the e-commerce project based on what would happen six months later - when the feature backlog will grow, and the team will expand. Most enterprise e-commerce platforms are complex product catalogs, with custom pricing rules, CMS integrations, role-based permissions, and multi-region deployments. They need discipline in the codebase - and in that context, Angular becomes an asset.
Why Angular Still Wins for Enterprise E-Commerce
Choosing Angular for ecommerce pays off as teams grow. Developers don’t have to reverse-engineer just to add a new widget. Angular’s structure keeps teams aligned - they move on by following the same patterns.
Routing, state management with RxJS, and native support for single‑page applications (SPA) are built in. Only the part of the screen that needs to change is refreshed, so users aren’t stuck watching a blank page load. Load‑on‑scroll catalogs, dynamic pricing updates, real‑time inventory - updates happen inside the existing page instead of forcing a full reload.
For mobile-first brands, Angular supports Progressive Web App features out of the box. You get offline support, and push notifications - without rewriting the app or maintaining a separate native version. Build PWAs because mobile cart abandonment drops when the interface behaves like a native app.
Server-Side Rendering (via Angular Universal) improves first-paint time, helps crawlers index product pages, and makes high-intent landing pages load. Angular doesn’t need extra plugins or community hacks to pull this off. Just run SSR for any e-commerce project with search functionality.
Component architecture is where Angular fits e-commerce best. Complex UIs - cart logic, account management, admin dashboards - benefit from reusable, isolated components. Teams don’t have to rebuild forms or filter menus every time. They extend, inject, and reuse.
Angular Front Ends Fit Into the Rest of Your Stack
Choosing Angular for the UI won’t disturb your existing technology landscape - it will integrate with it.
API
A custom e-commerce solution must integrate with various back-end systems and third-party services. Angular development approach is backend-agnostic and API-driven, enabling integration with any technology stack on the server side.
What that looks like in practice depends on the project. On one build, there may be a full MEAN stack (MongoDB, Express, Angular, Node) handling both sides of the integration. On others, Angular front-end may be connected to .NET or Java.
Whether it’s a legacy backend, a modern SaaS service, or something in between, the Angular UI can talk to it through REST or GraphQL endpoints.
Angular also works fluently with headless setups. If you’re running commercetools, BigCommerce, or Shopify Plus in API mode, Angular can pull in products, pricing, and promotions from the backend via exposed endpoints. The same applies for content: if a client is using Strapi or Contentful for CMS, developers can integrate those APIs into Angular components that render content dynamically.
Payments
Angular developers can build PCI-compliant integrations with Stripe, Braintree, PayPal, Authorize.net - wired directly into the UI, not patched in later. On mobile, they can use Stripe’s SDK for subscriptions and one-touch payments.
Shipping plus Fulfillment
Angular developers can connect Angular apps to FedEx, UPS, and USPS to automate shipping estimates, label printing, and tracking links. They can extend a QuickBooks integration to support additional carriers and plug in tools like EasyPost and ShipStation.
Marketing plus Analytics
Angular developers integrate tools like GA4, Mixpanel, or custom data layers depending on the analytics maturity of the client. Marketing workflows - email automation, CRM sync, campaign triggers - are configured through API connections to Mailchimp, Salesforce, or HubSpot.
ERP plus CRM Systems
Angular developers build integrations to SAP, Microsoft Dynamics, Oracle, and other enterprise systems through API. They configure mapping, transformations, and access controls - and when needed, build bridge services to keep things decoupled and maintainable.
Engineers define data flows and access patterns first, then build around API contracts and secure the data layer (OAuth, keys, and token management). If direct integration isn’t viable, they create middleware to buffer legacy constraints or orchestrate multi-system sync.
You get an Angular-based storefront that works with the way your business already runs - not a parallel system that becomes a pain to support.
Performance and Scalability in Angular E-Commerce
Angular makes promises about e-commerce platform performance. The moment when those promises get tested is when product pages spike under a campaign, when Black Friday traffic floods in, when mobile users scroll through dozens of variants and the site still responds in real time.
Angular apps can be designed to load only what’s needed. That means lazy loading feature modules so admin dashboards, account tools, or rarely used flows don’t ship with the initial bundle. In one Belitsoft's case, a coupon marketplace app tried to load 5,000 game records at startup. The result was obvious: stall, delay, drop-off. After refactoring, it loaded the first 10 and streamed the rest. The app felt immediate again.
Angular’s Ahead-of-Time (AOT) compilation pushes template rendering to the build step, so the browser has less work to do at runtime, resulting in faster load and render times. Tree shaking strips out dead imports. Combined with asset compression and image optimization, the result is lean.
Angular frontend is stateless by design. Once built, Angular apps behave like a brochure that’s downloaded once and then interacts with live services. That means horizontal scaling is simple: drop them behind a CDN or a cloud load balancer, and the site doesn’t care if you’re serving one user or one million. State lives in tokens or APIs. Nothing breaks under scale because nothing depends on a single server.
As an additional optimization step, Angular developers use SSR (Angular Universal) to push meaningful content to the screen before Angular even boots. The Node‑based server processes the first render. Angular takes over after. That gives fast initial paint, lower time-to-interactive, and lets crawlers see actual content - not a JavaScript shell. On high-traffic product pages, it means faster perception and better ranking. On mobile, it means fewer users bouncing before the app even starts.
They also split e-commerce platforms into functional modules: product catalog, user flows, cart and checkout, content integration. That modular architecture is a growth strategy to avoid technical debt before it becomes unavoidable.
With tools like NgRx and selective change detection, Angular keeps UI performance stable even when the app’s dealing with hundreds of SKUs, paginated search results, and persistent filters. Infinite scroll is engineered to avoid flooding the DOM.
Behind it all, deployment can be configured for growth too. CDNs cache what can be cached. API backends are cloud-hosted, with auto-scaling policies to cope with demand. If the store goes viral, the infrastructure flexes. If it’s a slow month, the cost stays low. No platform rewrites.
For the client, this means an Angular e-commerce store that not only works well on day one, but continues to deliver fast, reliable service as your customer traffic scales up.
Security in Angular E-commerce Projects
Front-End
Angular’s framework includes built-in defenses - automatic content sanitization, guarded templating, restricted DOM manipulation. Input is validated before it's sent, and user interactions are assumed untrusted by default. Form-level controls are set up to prevent broken flows or malformed payloads.
When dynamic HTML is unavoidable - say, rendering from a CMS - Angular developers use DomSanitizer, with context-specific rules. Components that could expose the client side to injection are reviewed individually.
Route access is locked down based on role. Angular guards is used to make admin screens inaccessible to unauthorized users. Sensitive states are tied to authentication flows, and nothing loads by default.
Authentication, Sessions, and Account-Level Security
Most apps rely on token-based authentication - either JWT or secure cookies, depending on the session model. Angular developers configure session storage and token expiration to balance user experience and risk. Admin logins, if present, are given additional controls: stricter timeouts, enforced 2FA, and audit trail requirements when requested.
When social login is used (Google, Facebook, etc.), the integration goes through the provider’s SDK with controlled scopes. Data transmission is encrypted by default - HTTPS is enforced from day one.
Back-End and Data Layer
Security on the server side covers the full stack. Input validation should be replicated - nothing should be trusted. SQL injection risks must be eliminated with parameterized queries, ORM protections, and manual review of dynamic operations. API endpoints must be protected by access control layers tied to role and context.
For payment flows, gateway integrations offload PCI scope. Special approaches are used to keep credit card data out of the app entirely. Tokenization is standard, and the app never stores cardholder data.
If sensitive data must be stored - for example, user PII or order history - it’s encrypted. Secrets are kept in vault services or injected securely at runtime. Credentials are never hard-coded or stored in repos.
Patching, and Ongoing Risk Management
Security reviews should be implemented in the code process. Angular and supporting libraries are patched on release. Angular team must track NPM packages and propose upgrade plans if a vulnerability affects the deployed app. Version pinning and package audits are part of the CI setup.
Compliance
For PCI DSS, the best practice is building to minimize scope - use payment provider infrastructure to capture card data, enforce password policies, and ensure no card details are written to disk. If the app needs to support transaction logging or access tracking, it must be built into the workflow.
For GDPR, consent flows should be implemented at page load: cookie banners, opt-ins, data erasure requests. If the app operates in the U.S., developers must adapt the same systems for CCPA - covering opt-out language and data access requests. This includes conditional activation of third-party scripts and restricting data sharing until user actions confirm consent.
Deployment and Infrastructure Practices
Good Angular development company also manages cloud infrastructure - firewall configuration, DDoS protection (when available through the cloud provider), and backup policies for data recovery. They advise on setup: TLS configuration, access control, API gateway policies.
Access credentials to hosting environments are kept in secure vaults. If access logs are required, Angular ecommerce developers work with the client’s operations team.
What Security Looks Like in Practice
No unauthenticated user can see internal data. No admin panel runs without access checks. No sensitive data is stored unless it’s encrypted. No payment flow runs outside a certified third-party process.
Belitsoft Uses Angular for E-Commerce
The team that turns Angular into a working e-commerce system
Belitsoft Angular developers don’t just know the syntax - they’ve solved actual e-commerce problems with it, across various industries, use cases, and complexity levels.
Take the gaming-enabled coupon marketplace they rebuilt. Originally built in AngularJS, the app needed a full migration to modern Angular - for performance and for feature expansion. The platform lets users form teams, play games, and unlock group discounts - a UX that combines shopping mechanics with multiplayer game logic. Belitsoft not only refactored the front-end into reusable Angular components, but also rebuilt the checkout flow for speed, stability, and lower bounce rates. Conversion went up, maintenance costs went down - that’s what clients actually want.
Belitsoft’s portfolio includes Angular-based e-commerce work for European retailers, including custom storefronts with integrated payment gateways and lead-gen from social channels. Our teams have built full-cycle platforms - from product pages to cart logic to payment confirmation - and optimized every step of the buying process.
Belitsoft runs with over 200 full-time specialists: Angular developers, QA engineers, product managers, and backend teams - all under one roof. Our Angular stack includes RxJS for reactive flows, NgRx/Redux for complex state management, and TypeScript across the board. They’re baseline tools when you’re running product filters, inventory logic, and user authentication - all on the same page, without reloads.
E-commerce punishes mistakes: slow load times, abandoned carts, broken mobile layouts. Belitsoft builds with that pressure in mind: cart UIs should adapt to screen size and session state, payment systems must actually work, product modules shouldn’t break when the inventory system hiccups.
In every Angular project, we're focused on checkout friction, search responsiveness, and mobile-first interaction. That’s how we raise cart completion: cleaner search filters, one-page checkout, faster product detail loads.
Belitsoft bakes in secure payment gateway integrations, fraud prevention steps, and compliance checks directly into the Angular codebase. It’s part of building a platform that survives in production.
Customization and Feature Development
One of the primary reasons companies opt for a custom-built e-commerce solution is the need for highly specialized features and unique user experiences. Belitsoft's development team builds complex, bespoke functionalities from scratch and tailor the product to exact business requirements. Whether you envision a 3D product configurator, a custom discount engine, an interactive storefront game, or a tailor-made analytics dashboard, Belitsoft has likely done something similar and can deliver it within an Angular framework.
For different clients, we have built interactive dashboards (using Angular’s charting libraries and real-time data binding) to visualize sales or user data, as well as custom product management interfaces with very specific workflows.
Belitsoft’s full-stack expertise means if it can be coded, we can incorporate it into the e-commerce platform. We often start with a requirements gathering and brainstorming. Belitsoft’s team includes both developers and business analysts who help translate ideas into technical specifications.
Angular’s component-based structure allows Belitsoft to develop new feature modules in isolation and then plug them into the overall application. If a feature is highly interactive (such as a product customizer where users pick options and see a live preview), Belitsoft uses Angular’s two-way data binding and possibly WebSocket integrations for real-time updates. If the feature requires heavy computation (like generating a custom price quote based on many parameters), we may implement part of it on the server.
Intuitive, brand-aligned UX/UI for the e-commerce platform
We either work with the client’s design team or provide their own UI/UX design services. Belitsoft has in-house designers. During the design phase, we gather the client’s branding guidelines, preferred color schemes, logos, and design ideas.
Belitsoft’s designers produce wireframes and prototypes for key screens like the home page, product pages, cart, and custom feature screens. Once the visual design is approved, the development team implements it in Angular, using Angular Material or custom CSS frameworks. We pay attention to responsive design so that the experience is consistent across devices. Belitsoft can also integrate a CMS for content management (managing homepage banners, blog content, etc.) so that the marketing team can easily update the UI text or images without developer intervention (for example, inserting a promo banner via a headless CMS that the Angular app pulls in).
Development Process and Collaboration
Most projects follow an Agile rhythm, usually two-week sprints. For fixed-scope projects - say, migrating a system with no moving parts - we can use waterfall.
Belitsoft assigns a project manager, sometimes paired with a business analyst. Clients can sit in on sprint reviews, give feedback during demos, or adjust priorities between cycles. Belitsoft works in Slack, Teams, Zoom, Jira - whatever the client uses.
On larger builds, especially ones touching complex domains or legacy systems, Belitsoft brings in a solution architect or domain-specific analyst. These roles work directly with client-side stakeholders to unpack high-level goals into smaller, technically scoped work.
Our QA engineers test manually and write automation scripts where coverage matters - front-end workflows, calculations, integration points. Test findings go into Jira, fixes move quickly.
Diagrams, interface descriptions, setup instructions - these come together alongside the code. If the client plans to maintain the system, Belitsoft provides walkthroughs. If not, we continue support - either as structured maintenance or as-needed troubleshooting.
The combination of technical prowess and focus on user-centric design enables Belitsoft to deliver e-commerce solutions - functionally rich, delightful to use and aligned with the client’s brand identity.
Cost, Timeline, and ROI
Belitsoft works closely with clients to ensure costs and timelines are well-estimated. At the start of a project, Belitsoft typically conducts a detailed discovery or estimation phase where requirements are clarified and features are prioritized. Based on the scope, we recommend a suitable engagement model - either Fixed-Price or Time & Materials (T&M), or even a Dedicated Team model.
Fixed-Price Model
Best suited for smaller projects or those with a well-defined, unchanging scope (for example, a straightforward e-commerce MVP or a short-term development sprint). In a fixed-price engagement, Belitsoft provides a detailed quotation up front, and commits to delivering the agreed scope within that budget and a mutually agreed timeline. This model gives the client cost certainty. Belitsoft ensures that all requirements are fully understood beforehand because changes mid-stream would require re-estimation. Typically, fixed-price projects have strictly determined deadlines and limited budgets. Belitsoft’s project planning in these cases is very thorough - we include a buffer for risk and ensure milestones are met to deliver on time. For example, a 3-month project to develop a custom Shopify-like MVP with Angular might be done fixed-price if all features are known.
Time & Materials
Ideal for medium to large projects, or those where scope may evolve (which is often true of custom e-commerce as user feedback or market changes can prompt new requirements). In T&M, the client pays for the actual effort (hours) expended, usually on a periodic basis. Belitsoft often uses T&M, where clients' priorities may shift each sprint. Features can be added or changed on the fly and the timeline can adapt as needed. We track time and provide transparency into how hours are used.
Dedicated Team / Cost-Plus
For ongoing development needs or very large-scale initiatives, Belitsoft can provide a dedicated team of developers, designers, QAs, etc., acting as an extension of the client’s own team. This is a variant of T&M, usually on a monthly rate per team member (plus a management fee). It’s suited for product companies or enterprises wanting to continuously develop and improve an e-commerce platform over years. This model is common when an in-house team needs augmentation for long-term projects
Post-Launch Support and Maintenance
Choosing Belitsoft for development means gaining a reliable support partner post-launch.
Your Angular e-commerce application will remain up-to-date, secure, and running at peak performance.
One specific aspect of maintenance is keeping the Angular framework and related libraries up-to-date. Belitsoft offers Angular upgrade services to migrate your application to the latest versions in a planned manner. When you have Belitsoft as your partner, we will monitor Angular releases (as well as updates to any integrated services or dependencies) and recommend when it’s time to upgrade. We often test the upgrade on a staging environment first, ensuring that all functionality remains intact.
With options for 24/7 support, proactive maintenance, and continuous improvement, Belitsoft covers the entire lifecycle of the application. This allows the client to focus on business operations and strategy, while Belitsoft takes care of keeping the technology in top shape. The peace of mind that comes from knowing experts are on standby to fix any problem and to enhance the platform over time is a significant benefit of partnering with Belitsoft for your custom e-commerce solution.
Rate this article
Recommended posts
Portfolio
Our Clients' Feedback
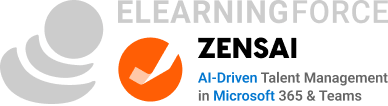
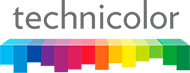
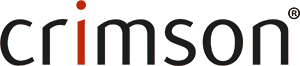
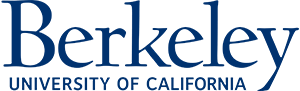



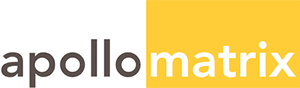
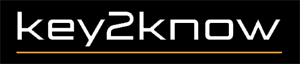
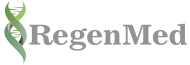
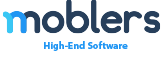

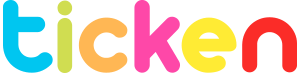
We have been working for over 10 years and they have become our long-term technology partner. Any software development, programming, or design needs we have had, Belitsoft company has always been able to handle this for us.
Founder from ZensAI (Microsoft)/ formerly Elearningforce