Executives making hiring, team composition, and skill-gap decisions need a practical guide for evaluating ASP.NET Core developers. This ASP.NET Core skillset evaluation framework benefits technical leaders, hiring managers, and talent acquisition professionals by providing a structured, comprehensive approach to assessing developer competency. Hiring managers can avoid costly mis-hires by using the framework’s detailed skill criteria, team leads can proactively close gaps, ensuring project success, and executives can ensure technical teams support enterprise priorities. Each section includes "proficiency indicators" that clearly specify what to look for when evaluating candidates.
General ASP.NET Core Platform Knowledge
To work effectively on ASP.NET Core open-source framework, developers need deep familiarity with the .NET runtime.
That starts with understanding the project layout and the application start-up sequence - almost every extensibility point hangs from those hooks.
Proficiency in modern C# features (async/await, LINQ, span-friendly memory management) is assumed, as is an appreciation for how the garbage collector behaves under load.
The day-to-day tool belt includes the cross-platform .NET CLI, allowing the same commands to scaffold, build and test projects.
A competent engineer can spin up a Web API, register services against interfaces, and flow those dependencies cleanly through controllers, background workers and middleware.
The resulting codebase stays loosely coupled and unit-testable, while the resulting Docker image deploys identically to Kubernetes or Azure App Service.
Essential skills include choosing the correct middleware order, applying async all the way down to avoid thread starvation, or swapping a mock implementation via DI for an integration test.
ASP.NET Core’s performance overhead is low, so bottlenecks surface in application logic rather than the framework itself. Mis-configurations, on the other hand, quickly lead to unscalable systems.
For the business, these skills translate directly to faster release cycles, fewer production incidents and “happier” operations dashboards.
When assessing talent, look for developers who can articulate how .NET differs from the legacy .NET Framework and who keep pace with each LTS release - such as adopting .NET 8’s minimal-API hosting model.
They should confidently discuss middleware ordering, demonstrate swapping concrete services for tests, and show they follow NuGet, async and memory-usage best practices. Those are the signals that a candidate can harness ASP.NET Core’s strengths.
Every ASP.NET Core developer we provide is evaluated using the same criteria - from runtime fundamentals to real-world middleware patterns - so you know exactly what you're getting before the work begins.
Web Development Paradigms with ASP.NET Core
On the server-side you can choose classic MVC - where Model, View and Controller are cleanly separated - or its leaner cousin Razor Pages, which combines view templates and handler logic together for page-centric development.
For service endpoints, the ASP.NET Core framework offers three gradations:
- full-featured REST controllers;
- gRPC for high-throughput internal calls;
- and the super-light Minimal APIs that strip the ceremony from micro-services.
When a use-case demands persistent client-side state or rich interactivity, you can reach for a Single-Page Application built with React, Angular or Vue - or stay entirely in .NET land with Blazor. And for real-time fan-out, SignalR pushes messages over WebSockets while falling back gracefully where browsers require it.
Choosing among these paradigms is largely a question of user experience, scalability targets, and team productivity.
SEO-sensitive storefronts benefit from MVC’s server-rendered markup. A mobile app or third-party integration calls for stateless REST endpoints that obey HTTP verbs and return clean JSON. Rich, internal dashboards feel snappier when the heavy lifting is pushed to a SPA or Blazor WebAssembly, while live-updating widgets - stock tickers, chat rooms, IoT telemetry - lean on SignalR to avoid polling. Minimal APIs shine where every millisecond and container megabyte counts, such as in micro-gateways or background webhooks.
Selecting the right model prevents over-engineering on the one hand and a sluggish user experience on the other.
From an enterprise perspective, fluency across these choices lets teams pick the tool that aligns best with maintainability and long-term performance.
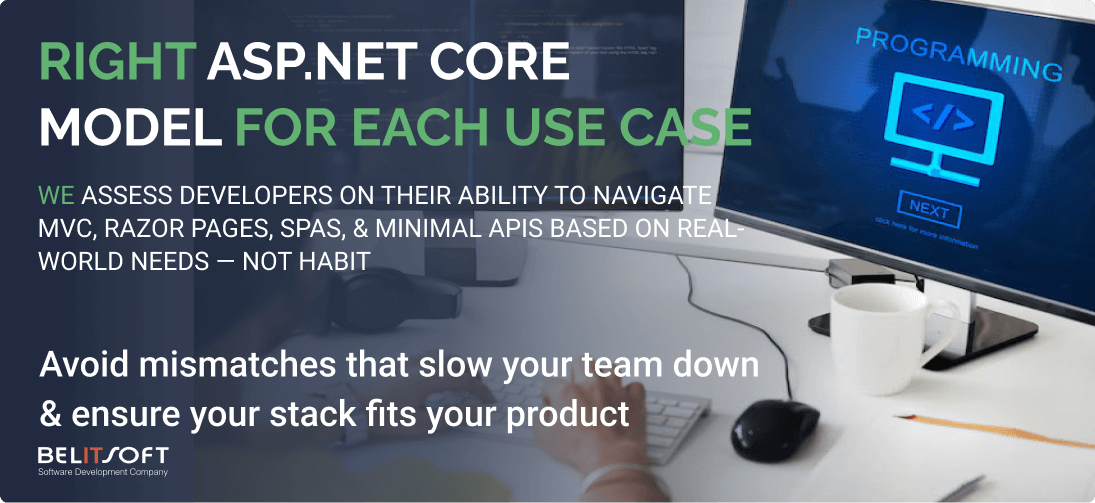
Hire candidates who can:
- wire up MVC from routing to view compilation;
- outline a stateless REST design with proper verbs, versioning and token auth;
- explain when Razor Pages beats MVC for simplicity;
- discuss Blazor and SignalR.
They won’t default to the wrong paradigm simply because it’s the only one they know.
Application Security in ASP.NET Core
Identity, OAuth 2.0, OpenID Connect and JWT bearer authentication give teams a menu of sign-in flows that range from simple cookie auth to full enterprise single sign-on with multifactor enforcement.
Once a user is authenticated (authN), a policy-based authorization (authZ) layer decides what they can do, whether that means “finance-report readers” or “admins with recent MFA.” Under the hood, the Data Protection API encrypts cookies and antiforgery tokens, while HTTPS redirection and HSTS can be flipped on with a single middleware - shutting the door on downgrade attacks.
Those platform primitives only pay off when paired with secure-coding discipline.
ASP.NET Core makes it easy - input validation helpers, built-in CSRF and XSS defenses, and first-class support for ORMs like Entity Framework Core that handle parameterized SQL - but developers still have to apply them consistently. Secrets never belong in source control - they live in user-secrets for local work and in cloud vaults (Azure Key Vault, AWS Secrets Manager, HashiCorp Vault) once the app ships.
Picture a real banking portal: users log in through OpenID Connect SSO backed by MFA, role policies fence off sensitive reports, every request travels over HTTPS with HSTS, and configuration settings (DB strings, API keys) sit in a vault. Each API issues and validates short-lived JWTs, while monitoring hooks, watch for anomalous traffic and lock out suspicious IPs.
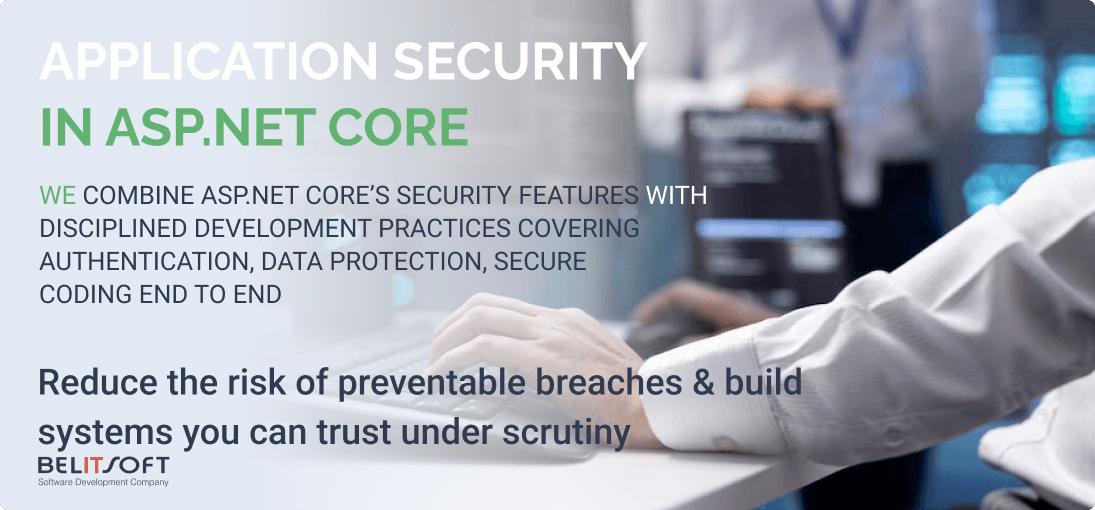
Assessing talent, therefore, means looking for engineers who can:
- wire up Identity or JWT auth and clearly separate authentication from authorization
- recite the OWASP Top Ten and show how ASP.NET Core’s built-ins mitigate them
- pick the right OAuth 2.0 / OIDC flow for a mobile client versus server-to-server
- encrypt data in transit and at rest, store secrets in a vault, stay current on package updates, enforce linters, and factor in compliance mandates, such as GDPR or PCI-DSS.
Those are the developers who treat security as a continuous practice, not a checklist at the end of a sprint.
ASP.NET Core Architectural Patterns
Early in a product’s life, you usually need speed of delivery more than anything else. A monolith - one codebase, one deployable unit - gets you there fastest because there’s only a single place to change, test, and ship. The downside appears later: every feature adds tighter coupling, builds take longer, and a single bug (or spike in load) can drag the whole system down. Left unchecked, the codebase turns into the dreaded "big ball of mud."
When that friction starts to hurt, teams often pivot to microservices. Here, each service aligns with an explicit business capability ("billing," "reporting," "notifications," etc.). Services talk over lightweight protocols - typically REST for request/response and an event bus for asynchronous messaging - so you can scale, deploy, or even rewrite one service without disturbing the rest.
ASP.NET Core is a natural fit: it’s cloud-ready, and container-friendly, so every microservice can live in its own Docker image and scale independently.
Regardless of whether the whole system is one process or a constellation of many, you still need internal structure.
Four variants - Layered, Clean, Onion, and Hexagonal - all enforce the same rule: business logic lives at the center (Domain), use-case orchestration around it (Application), and outer rings (Presentation and Infrastructure) depend inward only. Add standard patterns - Repository, Unit-of-Work, Factory, Strategy, Observer - to keep persistence, object creation, algorithms, and event handling tidy and testable.
For read-heavy or audit-critical workloads, you can overlay CQRS - using one model for updates (commands) and another for reads (queries) - so reporting doesn’t lock horns with writes. Couple that with an event-driven architecture (EDA): each command emits domain events that other services consume, enabling loose, real-time reactions (like billing finished → notification service sends invoice email).
Why it matters to the enterprise
Good architecture buys you scalability (scale what’s slow), fault isolation (one failure ≠ total outage), and evolutionary freedom (rewrite one slice at a time). Poor architecture does the opposite, chaining every new feature to yesterday’s shortcuts.
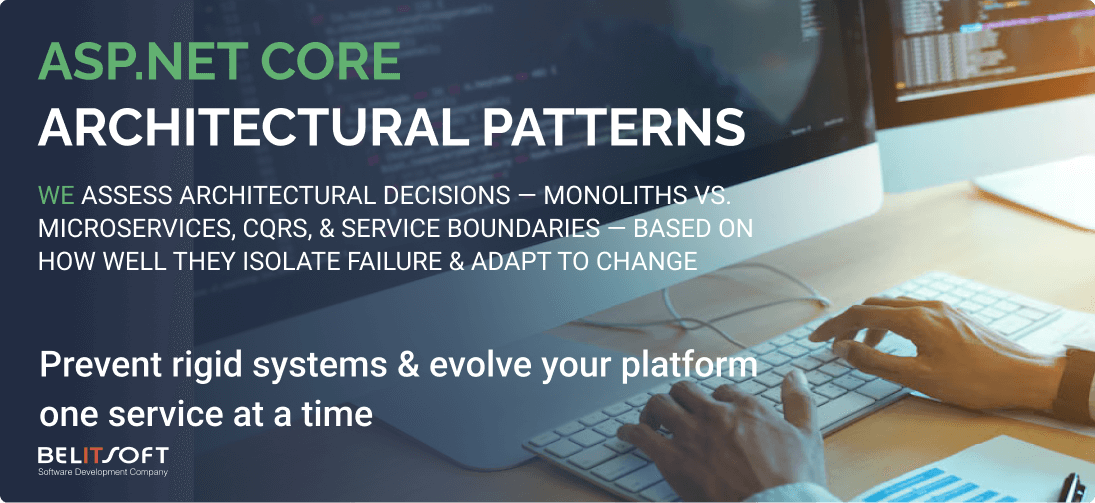
What to look for when assessing engineers
- Can they weigh monolith vs. microservices trade-offs?
- Do they apply SOLID principles and dependency injection beyond the basics?
- Do they explain and diagram Clean Architecture layers clearly?
- Have they implemented CQRS or event-driven solutions and can they discuss the pitfalls (data duplication, eventual consistency)?
- Most telling: can they sketch past systems from memory, showing how the pieces fit and how the design evolved?
A candidate who hits these notes is demonstrating the judgment needed to keep codebases healthy as systems - and teams - grow.
ASP.NET Core Data Management
A mature developer has deep proficiency in relational databases and Entity Framework Core: designing normalized schemas, mapping entities, writing expressive LINQ queries, and steering controlled evolution through migrations. They understand how navigation properties translate into joins, recognize scenarios that can still trigger N+1 issues, and know when to apply eager loading to avoid them. That is complemented by fluency with NoSQL engines (Cosmos DB, MongoDB) and high-throughput cache stores such as Redis, allowing them to choose the right persistence model for each workload.
The experienced engineer plans for hot-path reads by layering distributed or in-memory caching, tunes indexes, reads execution plans, and falls back to raw SQL or stored procedures when analytical queries outgrow ORMs. They wrap critical operations in ACID transactions, apply optimistic concurrency (row-versioning) to avoid lost updates, and always parameterize inputs to shut the door on injection attacks. Encryption - both at rest and in transit - and fine-grained permission models round out a security-first posture.
Picture an HR platform: EF Core loads employee-to-department relationships to keep the UI snappy, while heavyweight payroll reports are managed by a dedicated reporting service that runs optimized queries outside the ORM when needed. A Redis layer serves static reference data in microseconds, and read-replicas or partitioned collections absorb seasonal load spikes. Automated migrations and seed scripts keep every environment in sync.
For the enterprise, disciplined data management eliminates the slow-query bottlenecks that frustrate users, cuts infrastructure costs, and upholds regulatory mandates such as GDPR. Well-governed data pipelines also unlock reliable analytics, letting the business trust its numbers.
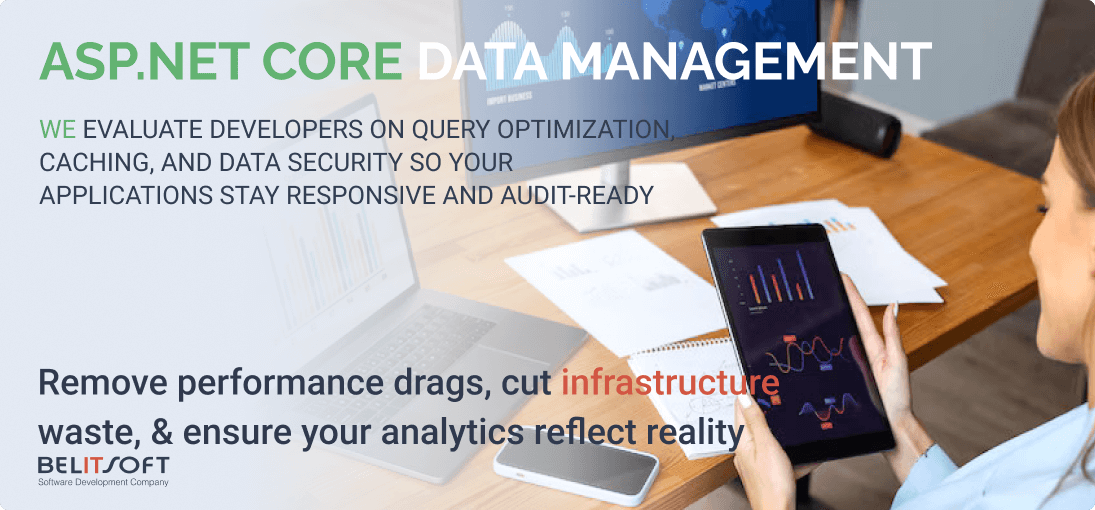
What to look for when assessing this competency
- Can the candidate optimize EF Core queries with .AsNoTracking, server-side filtering, and projection?
- Do they write performant SQL and interpret execution plans to justify index choices?
- Have they designed cache-invalidation strategies that prevent stale reads?
- Can they articulate when a document or key-value store is a better fit than a relational model?
- Do their code samples show consistent use of transactions, versioning, encryption, and parameterized queries?
ASP.NET Core Front-End Integration
Modern enterprise UIs are frequently built as separate single-page or multi-page applications, while ASP.NET Core acts as the secure, performant API layer. Developers therefore need a working command of both sides of the contract:
- Produce and maintain REST or gRPC endpoints.
- Manage CORS so browsers can call those endpoints safely.
- Understand HTML + CSS + JavaScript basics - even on server-rendered Razor Pages.
- Host or proxy compiled Angular/React/Vue assets behind the same origin, or serve them from a CDN while keeping API paths versionable.
- Leverage Blazor (Server or WebAssembly) when a C#-to-browser stack simplifies team skill-sets or sharing domain models.
- Document and version the API surface with OpenAPI/Swagger, tune it for paging, filtering, compression, and caching.
- Ensure authentication tokens (JWT, cookie, BFF, or SPA refresh-token flows) move predictably between client and server. Enable SSR or response compression when required by Core Web Vitals.
Real-world illustration
A production Angular build is copied into wwwroot and served by ASP.NET Core behind a reverse-proxy. Environment variables instruct Angular to hit /api/v2/. CORS rules allow only that origin in staging, and the API returns 4xx/5xx codes the UI maps directly to toast messages. A small internal admin site uses Razor Pages for CRUD because it can be delivered in days. Later, the same team spins up a Blazor WebAssembly module to embed a complex charting dashboard while sharing C# DTOs with the API.
Enterprise importance
A single misconfigured CORS header, token expiry, or uncompressed 4 MB payload can sabotage uptime or customer satisfaction. Back-end developers who speak the front-end’s language shorten feedback loops and unblock UI teams instead of becoming blockers themselves.
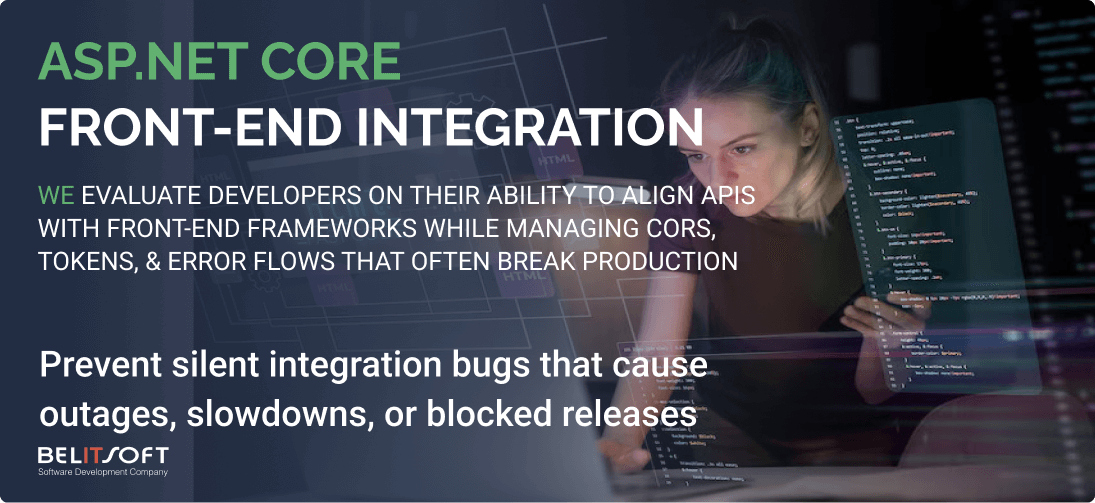
Proficiency indicators
- Designs REST or gRPC services that are discoverable (Swagger UI), sensibly versioned (/v1/, media-type, or header-based), and performance-tuned (OData-style querying, gzip/brotli enabled).
- Sets up AddCors() and middleware so that preflight checks, credentials, and custom headers all behave in pre-prod and prod.
- Has personally written or debugged JavaScript fetch/Axios code, so they recognise subtle issues like missing await or improper Content-Type.
Experiments with Blazor, MAUI Blazor Hybrid, or Uno Platform to stay current on C#-centric front ends. - Profiles payload size, turns on response caching, or chooses server-side rendering when TTI (Time to Interactive) must be under a marketing SLA.
ASP.NET Core Front-End Middleware
When an ASP.NET Core application boots, Kestrel accepts the HTTP request and feeds it into a middleware-based request pipeline. Each middleware component decides whether to handle the request, modify it, short-circuit it, or pass it onward. The order in which these components are registered is therefore critical: security, performance, and stability all hinge on that sequence.
Pipeline Mechanics
ASP.NET Core supplies a rich catalog of built-in middleware - Static Files, Routing, Authentication, Authorization, Exception Handling, CORS, Response Compression, Caching, Health Checks, and more. Developers can slot their own custom middleware anywhere in the chain to address cross-cutting concerns such as request timing, header validation, or feature flags. Because each middleware receives HttpContext, authors have fine-grained control over both the request and the response.
Dependency-Injection Lifetimes
Behind the scenes, every middleware that needs services relies on ASP.NET Core’s built-in Dependency Injection (DI) container. Choosing the correct lifetime is essential:
- Transient – created every time they are requested.
- Scoped – one instance per HTTP request.
- Singleton – one instance for the entire application.
Misalignments (like resolving a scoped service from a singleton) quickly surface as runtime errors - an easy litmus test of a developer’s DI proficiency.
Configuration & Options
Settings flow from appsettings.json, environment variables, and user secrets into strongly-typed Options objects via IOptions
Logging Abstraction
The Microsoft.Extensions.Logging facade routes log events to any configured provider: console, debug window, Serilog sinks, Application Insights, or a third-party service. Structured logging, correlation IDs, and environment-specific output levels differentiate a mature setup from “it compiles” demos.
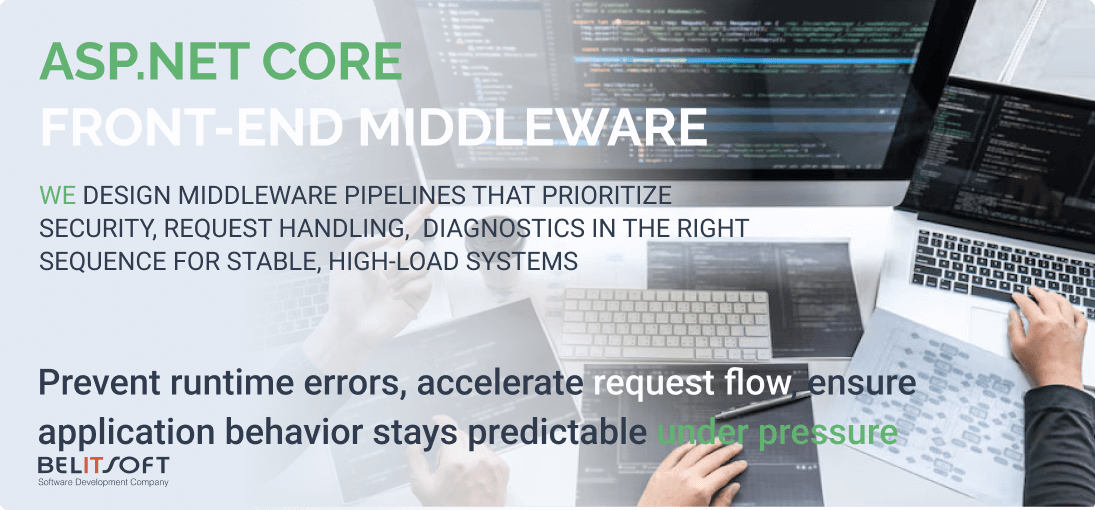
Practical Pipeline Composition
A developer who has internalized the rules will:
- Register UseStaticFiles() first, so images/CSS bypass heavy processing.
- Insert UseResponseCompression() (like Gzip) immediately after static files to shrink dynamic payloads.
- Place UseAuthentication() before UseAuthorization(), guaranteeing identity is established before policies are enforced.
- Toggle the Developer Exception Page in dev, while delegating to a generic error handler and centralized logging in prod.
- Insert bespoke middleware - say, a timer that logs duration to ILogger - precisely where insight is most valuable.
Enterprise Significance
Correctly ordered middleware secures routes, improves throughput, and shields users from unhandled faults - advantages that compound at enterprise scale. Built-ins accelerate delivery because teams reuse battle-tested components instead of reinventing them, keeping solutions consistent across microservices and teams.
When these mechanics are orchestrated correctly, the payoff is tangible: payloads shrink, latency drops, CORS errors disappear, compliance audits pass, and on-call engineers sleep soundly. Misplace one middleware, however - say, apply CORS after the endpoint has already executed - and the application may leak data or collapse under its own 403s.
Skill-Assessment Cues
Interviewers (or self-assessors) look for concrete evidence:
- Can the candidate sketch the full request journey - from Kestrel through each middleware to the endpoint?
Do they name real built-in middleware and explain why order matters? - Have they authored custom middleware leveraging HttpContext?
- Do they register services with lifetimes that avoid the scoped-from-singleton pitfall?
- Can they configure multi-environment settings and wire up structured, provider-agnostic logging?
A developer who demonstrates mastery of the foundational moving parts in ASP.NET Core is equipped to architect resilient, high-performance web APIs or MVC applications.
ASP.NET Core DevOps
Effective deployment of an ASP.NET Core application begins with understanding its hosting choices.
On Windows, the framework typically runs behind IIS, while on Linux it’s hosted by Kestrel and fronted by Nginx or Apache - either model can also be containerised and orchestrated in Docker.
These containers (or traditional processes) can be delivered to cloud targets - Azure App Service, Azure Kubernetes Service (AKS), AWS services, serverless Functions - or to classic on-premises servers. Whatever the venue, production traffic is normally routed through a reverse proxy or load balancer for resilience and SSL termination.
Developers bake portability in from the start by writing multi-stage Dockerfiles that compile, publish and package the app into slim runtime images. A continuous-integration pipeline - implemented with GitHub Actions, Azure DevOps, Jenkins or TeamCity - then automates every step: restoring NuGet packages, building, running unit tests, building the container image, pushing it to a registry and triggering deployment.
Infrastructure is created the same way: Infrastructure-as-Code scripts (Terraform, ARM or Bicep) spin up identical environments on demand, eliminating configuration drift. After deployment, Application Performance Monitoring tools such as Azure Application Insights collect request rates, latency and exceptions, while container and host logs remain at developers’ fingertips. Each environment (dev, test, staging, prod) reads its own connection strings and secrets from injected environment variables or a secrets store.
A typical cloud path might look like this: a commit kicks off the pipeline, which builds and tests the code, bakes a Docker image, and rolls it to AKS. A blue-green or staging-slot swap releases the new version with zero downtime. For organizations that still rely on on-premises Windows servers, WebDeploy or PowerShell scripts push artifacts to IIS, accompanied by a correctly-tuned web.config that loads the ASP.NET Core module.
The business result is a repeatable, script-driven deployment process that slashes manual errors, accelerates release cadence and scales elastically with demand.
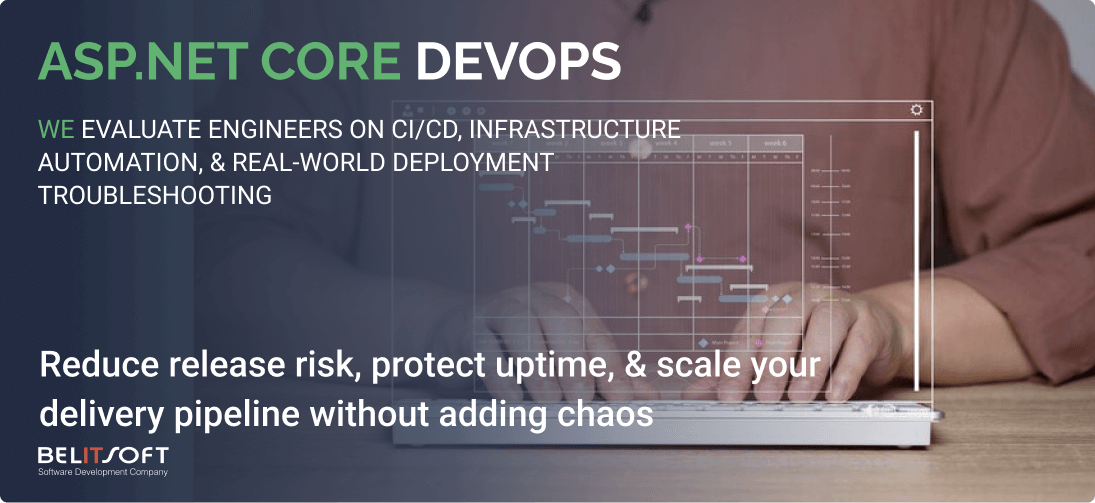
When assessing skills, look for engineers who:
- Speaks fluently about a real CI/CD setup (tool names, stages, artifacts).
- Differentiates IIS module quirks from straight-Kestrel Linux hosting and container tweaks.
- Diagnoses environment-specific failures - stale config, port bindings, SELinux, etc.
- Bakes health checks, alerts, and dashboards into every deployment.
- Writes IaC scripts and documentation so any teammate - or pipeline - can rebuild the stack from scratch.
A practitioner who checks these boxes turns deployment into a repeatable, push-button routine - one that the business can rely on release after release.
ASP.NET Core Quality Assurance
Quality assurance in an ASP.NET Core project is less a checklist of tools than a continuous story that begins the moment a feature is conceived and ends only when real-world use confirms the application’s resilience.
It usually starts in the red-green-refactor rhythm of test-driven development (TDD). Developers write unit tests with xUnit, NUnit or MSTest, lean on Moq (or another mocking framework) to isolate dependencies, and let the initial failures (“red”) guide their work. As code turns “green,” the same suite becomes a safety net for every future refactor. Where behavior spans components, integration tests built with WebApplicationFactory and an EF Core In-Memory database verify that controllers, middleware and data access layers collaborate correctly.
When something breaks - or, better, before users notice a break - structured logging and global exception-handling middleware capture stack traces, correlation IDs and friendly error messages. A developer skims the log, reproduces the problem with a failing unit test, and opens Visual Studio or VS Code to step through the offending path. From there they might:
- Attach a profiler (dotTrace, PerfView, or Visual Studio’s built-in tools) to spot memory churn or a slow SQL query.
- Spin up Application Performance Monitoring (APM) dashboards to see whether the issue surfaces only under real-world concurrency.
- Pull a crash dump into a remote debugging session when the fault occurs only on a staging or production host.
Fixes graduate through the pipeline with new or updated tests, static analysis gates in SonarQube, and a mandatory peer review - each step shrinking the chance that today’s patch becomes tomorrow’s outage.
Occasionally the culprit is performance rather than correctness. A profiler highlights the hottest code path during a peak-traffic window; the query is refactored or indexed, rerun under a load test, and the bottleneck closes. The revised build ships automatically, backed by the same green test wall that shielded earlier releases.
Well-tested services slash downtime and let teams refactor. Organizations that pair automated coverage with debugging shorten incidents and protect brand reputation.
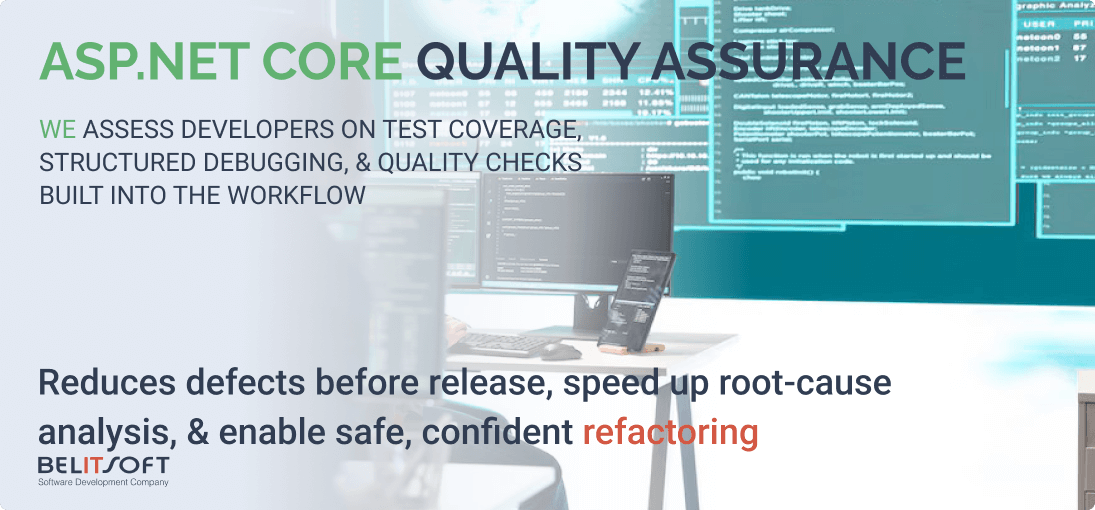
Interviewers and leads look for developers who:
- Write comprehensive unit and integration tests (and can quote coverage numbers).
- Spin up Selenium or Playwright suites when UI risk matters.
- Debug methodically - logs → breakpoint → dump.
- Apply structured logging, correlation IDs, alerting from day one.
- Implement peer reviews and static analysis.
How Belitsoft Can Help
Belitsoft is the partner that turns ASP.NET Core into production-grade, secure, cloud-native software. We embed cross-functional .NET teams that architect, code, test, containerize and operate your product - so you release faster and scale safely. Our senior C# engineers apply .NET tools, scaffold APIs, design for DI & unit-testing, and deliver container-ready builds.
Web Development
We provide solution architects that select the right paradigm up-front, build REST, gRPC or real-time hubs that match UX and performance targets.
Application Security
Our company implements Identity / OAuth2 / OIDC flows, policy-based authZ, secrets-in-vault, HTTPS + HSTS by default, automated dependency scanning & compliance reporting.
Architectural Patterns
Belitsoft engineers deliver Clean / Onion-architecture templates, DDD workshops, micro-service road-maps, event-bus scaffolding, and incremental decomposition plans.
Data Management
We optimize EF Core queries, design schemas & indexes, add Redis/L2 caches, introduce Cosmos/Mongo where it saves cost, and wrap migrations into CI.
Front-End Integration
Our developers expose discoverable REST/gRPC endpoints, wire CORS correctly, automate Swagger/OpenAPI docs, and align auth flows with Angular/React/Vue or Blazor teams.
Middleware & Observability
Belitsoft experts can re-order pipeline for security ➜ routing ➜ compression, inject custom middleware for timing & feature flags, and set up structured logging with correlation IDs.
DevOps & CI/CD
We apply TDD with xUnit/MSTest, spin up WebApplicationFactory integration suites, add load tests & profilers to the pipeline, and surface metrics in dashboards.
Looking for proven .NET engineers? We carefully select ASP.NET Core and MVC developers who are proficient across the broader .NET ecosystem - from cloud-ready architecture to performance-tuned APIs and secure, scalable deployments.Contact our experts.
Recommended posts
Portfolio
Our Clients' Feedback
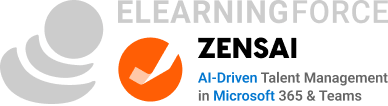
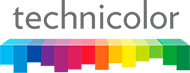
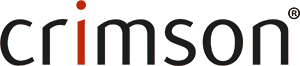
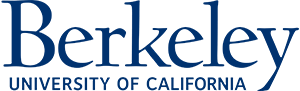



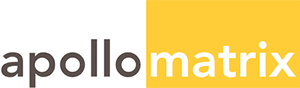
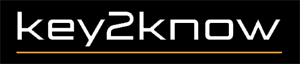
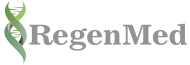
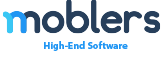

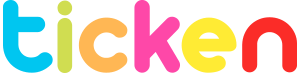
We have been working for over 10 years and they have become our long-term technology partner. Any software development, programming, or design needs we have had, Belitsoft company has always been able to handle this for us.
Founder from ZensAI (Microsoft)/ formerly Elearningforce